Changing to a new orbit or determining the maneuvers to rendezvous with another object can be difficult to accomplish manually. Gravity Engine provides a component to handle this type of “orbital path finding”: TransferShip. This component supports a wide variety of transfer types:
- Hohmann: a transfer from any circular orbit to another circular orbit. Supports cases where orbits differ in radius, inclination and location of ascending node.
- Hohmann – Rendezvous: rendezvous from a circular orbit to any other circular orbit (including the same orbit with different phase). Supports cases where orbits differ in radius, inclination and location of ascending node.
- Lambert Point: Determine a transfer to the specified point given a specific transfer time
- Lambert Orbit: Determine a transfer to a destination orbit at a designated phase in a given transfer time
- Lambert Intercept: Transfer to intercept a target object in the specified time
- Lambert Rendezvous: Transfer to rendezvous with a designated object in a specified time.
Gravity Engine provides two scenes that demonstrate the use of TransferShip in the Scenes/MiniGames/Scenes/InOrbit-Transfers directory
- TransferShip: a bare-bones demonstration of the capability
- TransferShipWithOrbitPoint: A full-featured controller than allows a transfer to be specified at a future point and shows the transfer path that will be taken in advance.
The operation of both scenes is provided in the following video:
Orbit Transfer Types
Hohmann transfer refer to a transfer from/to a circular orbit. This transfer is almost always the lowest delta-V method for the transfer. The transfer time is predetermined by the algorithm and corresponds to the time required for half an orbit with a semi-major axis that is the average of the from/to orbit semi-major axes. In the case of a rendezvous the start of the transfer may be delayed and an intermediate phasing orbit may be employed to ensure the rendezvous. This will result in a longer transfer time. (In some cases a bi-elliptic transfer may be the optimal dV transfer. These transfers are typically very slow and are currently not considered in the TransferShip logic).
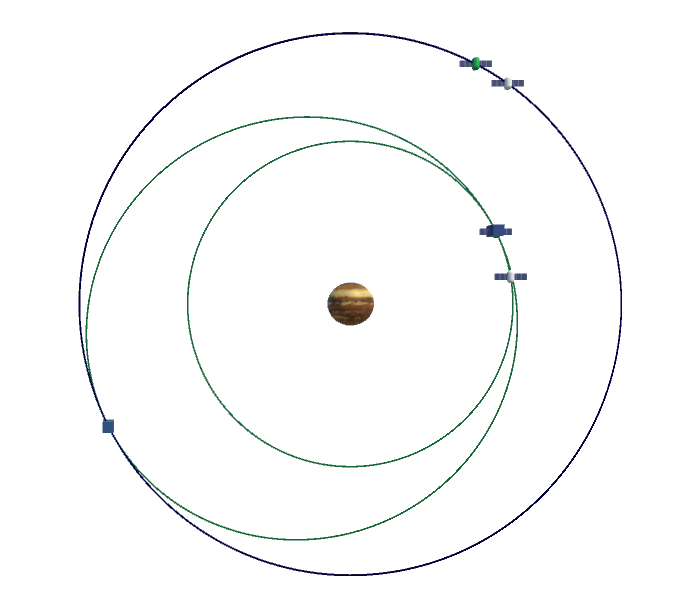
A Lambert transfer is a direct path from the start point to the designated destination with a specified transfer time. The path may be elliptical or hyperbolic depending on the details of the transfer. A Lambert transfer may be used to/from circular orbits if control over the time of the transfer is required. The Lambert transfer logic ensures that the direction of the transfer does not result in a reversal of the orbital motion.
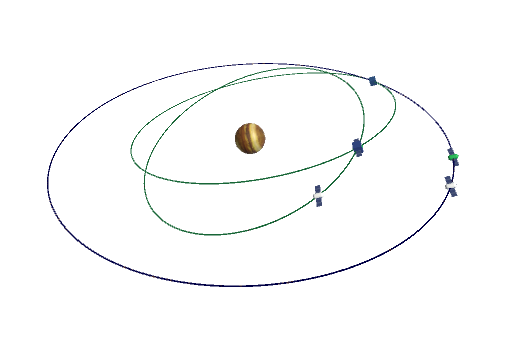
TransferShip Component
The TransferShip component is attached to the NBody object that will perform the transfer. This component does not have any direct UI interaction. The transfer is triggered by a call to the DoTransfer() method by control code in the scene.
TransferShip assumes that the NBody object it is attached to has a child game object with an OrbitPredictor component. This is used to determine the initial conditions of the orbit transfer.
The inspector view of this component is:
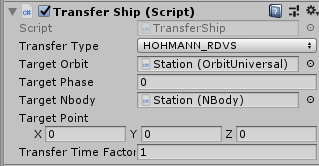
- Transfer Type:
- HOHMANN: Immediate transfer from one circular orbit to circular orbit specified by Target Orbit
- HOHMANN_RDVS: Transfer from one circular orbit to another with phasing so arrival matches location of targetNBody
- LAMBERT_POINT: transfer to the Target Point using the Transfer Time Factor
- LAMBERT_ORBIT: transfer to the Target Phase in the Target Orbit using the Transfer Time Factor
- LAMBERT_RDVS: rendezvous with the target NBody using the Transfer Time Factor. Match the orbit on arrival.
- LAMBERT_INTERCEPT: intercept the target NBody using the Transfer Time Factor. Do not match the target orbit on arrival.
- Target Orbit: An OrbitUniversal that defines the transfer destination
- Target Phase: The position in the target orbit of the destination when LAMBERT_ORBIT is used
- Target NBody: the target NBody when a rendezvous or intercept transfer type is selected
- Target Point: the destination point used with the LAMBERT_POINT transfer type
- Transfer Time Factor: The Lambert transfer time, relative to the minimum energy transfer time for the scenario chosen
TransferShip Implementation
This component manages the interactions with the core GE classes that determine transfers based on the selected transfer type. All Hohmann transfers make use of the HohmannGeneral class. This class replaces the several special case classes that were defined in earlier versions of GE (these are still provided to ensure backwards compatibility). The TransferShip controller makes use of LambertUniversal for the Lambert transfers. The specific API calls vary depending on the type of Lambert transfer that has been selected. The specific code is in the ComputeLambert() method in TransferShip.
Transfer Ship Scene
The transfer ship scene uses a very simple controller: TransferShipController. This controller invokes the DoTransfer() method when the X key is pressed. The scene does not show the orbit transfer in advance.
Transfer Ship At Orbit Point Scene
This scene provides a full featured orbit path preview from a point on the ship orbit. The point for the transfer start can be adjusted with player mouse input. In Lambert transfer modes the destination point and time of flight can also be adjusted with player mouse input.
Overview
The ability to perform a transfer at a future point in the orbit is accomplished by having “ghost” ship and target objects in the scene (SpaceshipAtOrbitPoint and StationAtTargetPoint). When a maneuver is selected these objects are made active and added to GE. The type of transfer for the maneuver is specified by the TransferShip component attached to the ghost ship. The SpaceshipAtOrbitPoint position is under the control of the OrbitPoint component. In the scene provided this is set to be of type PHASE_FROM_MOUSE allowing the player interactive control over the location at which the transfer calculation will start. As the phase of the start point is moved, the StationAtOrbitPoint will be adjusted to be in the position that corresponds to the time required to get to the start point.
The keys W/S are used to adjust the relative transfer time for the LAMBERT mode transfers. The default transfer time is determined by the Lambert minimum energy path.
When the maneuver planning is active, the controller uses the TransferShip element on the SpaceshipAtOrbitPoint to determine the maneuvers required for the transfer. The DisplayManeuvers component on the TransferSceneController object is then used to illustrate the resulting orbits for the transfer and the points at which the maneuvers will occur. This allows the player to see a complete preview of the maneuver.
Implementation
The code for the transfer is located in the TransferShip component attached to the ghost ship, SpaceshipAtOrbitPoint. This is initially inactive in the scene to ensure GE does not add it at start. The TransferShip is configured to use the StationAtOrbitPoint as the target for intercept and rendezvous maneuvers. The ghost ship has an orbit point that is used to position the ghost ship. This OrbitPoint has direct mouse control disabled; the mouse input is first checked in the TransferSceneController and passed on to the OrbitPoint when that controller has determined that is the appropriate handler.
The TransferSceneController has simple state machine that cycles through IDLE, SET_<X> (X is POINT, LAMBERT or HOHMANN) and EXECUTE_MANEUVER. Depending on the requested transfer there may be up to 3 maneuvers to accomplish the transfer. The display of these is handled by the DisplayManuevers component (see below).
When the user presses M the state machine advances to the SET_<X> state that corresponds to the type of orbit set in the TransferShip component. Different states are required since different user input applies. In the Lambert transfers the time of the transfer can be adjusted with mouse input. Hohmann transfers have a transfer time determined by the to/from orbit geometries.
Determination of the maneuvers required in handled by a call to transferShip.ShipMoved(). This cause the transfer ship to determine the series of maneuvers required for the transfer. The controller then passes this list of maneuvers to the DisplayManeuvers component.
Displaying Maneuvers
The DisplayManuver component is attached to the TransferSceneController game object. It is detected automatically by the controller script. The inspector view of this component is:
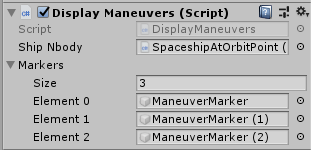
The ship NBody makes reference to the ship that has the maneuvers to be displayed. Each maneuver in the sequence is displayed using the corresponding marker in the list of markers. These markers are inactive game objects attached to the TransferSceneController. Each maneuver marker has an NBody, an OrbitPredictor and some visual object (e.g. cube).
DisplayManuevers is passed a list of maneuvers by the TransferSceneController. It makes use of the information in the maneuvers to place each marker at the correct location with the velocity that will result from the maneuver. This allows the OrbitPredictor component to show that portion of the maneuver.
Executing the Transfer
Pressing the X key executes the transfer. This results in a call to transferShip.DoTransfer(). The state logic then moves on to the EXECUTE_MANUEVER state. The controller adds a callback to the final maneuvers as part of the call to DoTransfer(). This ensures the controller is aware of when the final maneuver is executed, allowing it to return to the IDLE state.