Tutorial: Particles
Contents
Tutorial: Particles¶
Particles from a Unity particle system can also be moved by GE2. Unity provides a lot of control over particle generation and lifetimes
giving them “a life of their own”. This requires tight coupling between the gravitational force calculations and code that checks
for particle creation/extinction. Particles can be created by the Unity using the standard Unity component ParticleSystem
. If the same
game object with the particle system has a GSParticles
component then GE2 will handle the movement of the particles. Additional components
can be added to manage the initial conditions of the particles. For example DustRing
initializes particles in an orbit around a central body
and DustBall
creates a ball of particles with a specific world space position and velocity.
The evolution of particles is tightly linked to a specific display context and particle system so particles must be added to a GSDisplay
managed object. This precludes having two views
of the same exact particle positions. If a series of small objects in two views is required that is best done by adding them as massless bodies using GSBody
.
Currently the evolution of the particles is done on the main thread (not job mode) independent of the choice of EvolveMode
in the GSController
. The eventual goal is to remove this
limitation after more debugging (there were issues with particle spawning that were set aside for now).
The tutorial scene presents two GE2 particle components: DustBall
and DustRing
.
DustBall¶
The DustBall
component provides the initialization for a set of GSParticles
. The following components are required to instantiate an instance:
ParticleSystem
(describes the number and size of particles in the usual way)GSParticles
(indicates to GE2 that these particles will be evolved by aGSController
)DustBall
(provides an inspector to indicate the size and initial velocity of the ball of particles)
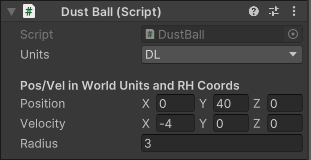
DustRing¶
Particles may also be placed in an orbital ring around a gravitational body using the DustRing
component. Like the dust ball example, three components are required:
ParticleSystem
(describes the number and size of particles in the usual way)GSParticles
(indicates to GE2 that these particles will be evolved by aGSController
)DustRing
(provides an inspector to indicate the orbital elements of the particle ring)
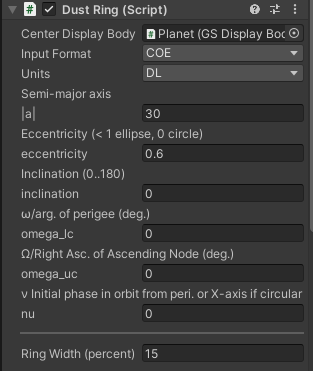
The orbital elements are defined in the same way as for a GSBody
. The width of the ring is defined as a percentage of the orbital radius of the ring.
Implementation Notes¶
Implementing gravitational motion for particles while using the standard Unity ParticleSystem
for particle generation, extinction and rendering is a bit tricky. This
is handled by the GSParticles
component. This component has resposibility for:
monitoring the particle system for the creation/removal of particles
evolution of the particles under the influence of all masses in the system
The evolution of particles is done within each GSParticles
instance. This is the one case in which the gravitational evolution is not handled by GECore
and GEPhysicsJob
.
Currently the evolution of particles always happens on the update cycle. There is some code under development to have this done using the job system but this is not
currently “wired in”.